Personal Site
Personal
In order of expanding my technical knowledge, I wanted to development a new personal website that served as both a portfolio showcase and a learning playground. This project allowed me to deepen my knowledge across multiple technologies and concepts while implementing professional-grade solution to my own use.
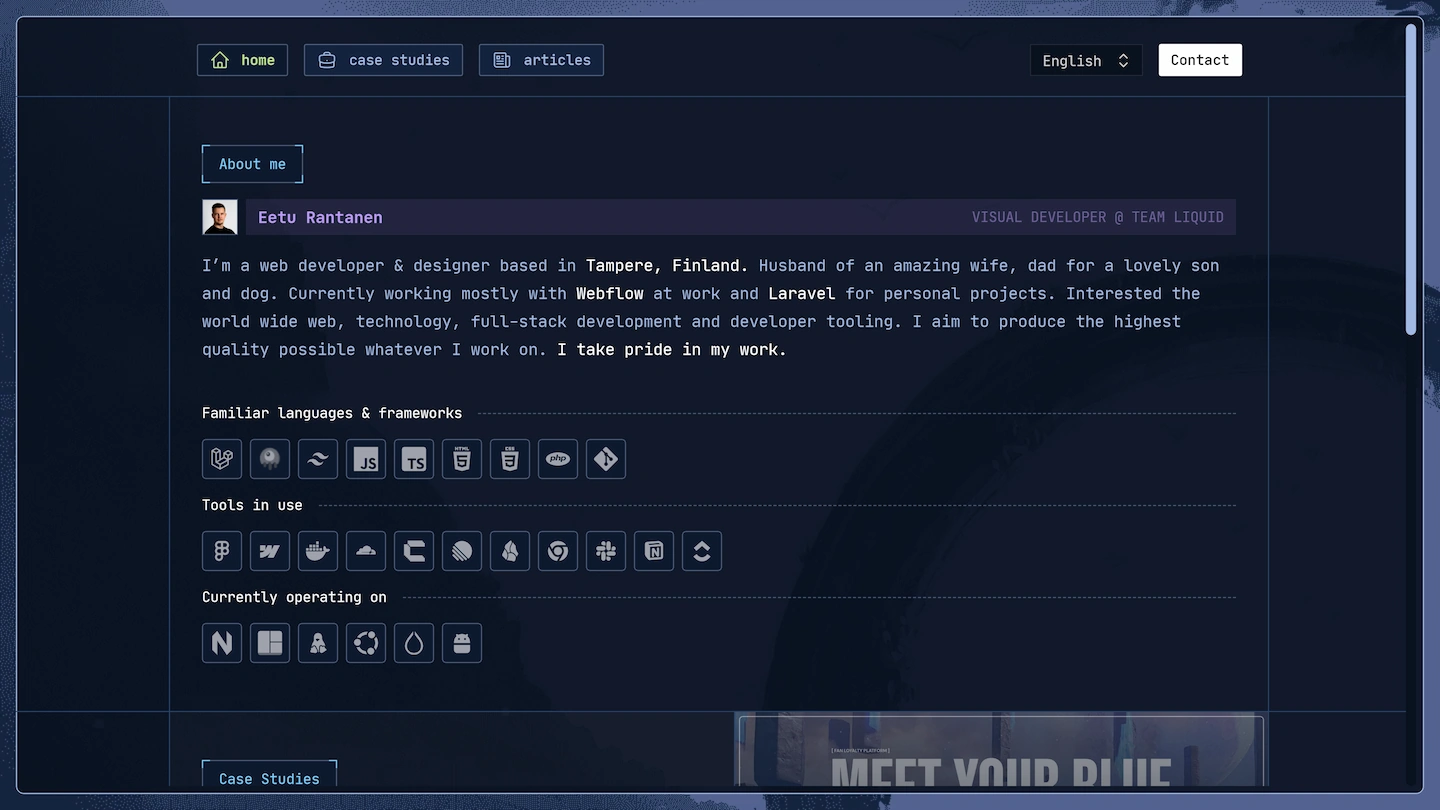
In order of expanding my technical knowledge, I wanted to development a new personal website that served as both a portfolio showcase and a learning playground. This project allowed me to deepen my knowledge across multiple technologies and concepts while implementing professional-grade solution to my own use.
Technical Implementation
Laravel, Livewire, SQLite and Filament
The core of my website is built on Laravel, Livewire, SQLite and Filament. This combination allowed me to create a responsive, dynamic site with sophisticated admin capabilities. SQLite as an embedded database ensures very fast data fetching.
Laravel Implementations
Queue System Implementation I configured Laravel's queue system to handle resource-intensive operations asynchronously, significantly improving user experience by:
- Handling email dispatches without blocking user requests
- Scheduling periodic tasks like database backups and analytics reporting
Mailing Automation I implemented an email system that includes:
- Contact form submissions to my email inbox
- Templated emails with branded styling using markdown.
- Email queue management to prevent sending failures
// This is how I handle form submissions. Send mail to myself
// and put the user submission to queue so they can continue using the site.
public function send()
{
$this->validate();
$mailData = Contact::create([
'first_name' => $this->firstName,
'last_name' => $this->lastName,
'company' => $this->company,
'email' => $this->email,
'message' => $this->message,
]);
Mail::to('eeturantanen.dev@gmail.com')
->queue(new ContactEmail($mailData));
$this->reset();
Flux::toast(
variant: 'success',
heading: 'Your message has been sent!',
text: 'Thanks for reaching out! 🙂'
);
}
Internationalization & Localization I built a fully translatable interface using Laravel's localization features:
- Implemented language switcher
- Created translation files for English and Finnish.
// This is how I handle chaning the language with the dropdown
public function setLocale(string $locale)
{
if (in_array($locale, config('app.available_locales'))) {
App::setLocale($locale);
Session::put('locale', $locale);
$this->currentLocale = $locale;
$this->dispatch('language-changed'); // Emit event for other components
$this->redirect(url()->previous(), navigate: true);
}
}
Content Management with Filament
I constructed a custom CMS using Filament that gives me complete control over site content:
- Created custom resource panels for case-studies, articles, tags and work samples.
- Implemented authorization to the admin panel
- Built custom field types for specialized content needs
- Added media management with previews and organization features
- Developed custom validation rules to maintain content quality
Cloud Storage Integration
Cloudflare R2 Implementation I established a seamless cloud storage solution using Cloudflare R2:
- Configured Laravel's filesystem to use R2 as a storage disk for asset serving and database backups
- Created automated image uploading from the CMS with proper validation
- Set up caching policies to improve performance and reduce bandwidth usage
To make R2 work with Filament, I need to patch the
'Filament\Forms\src\Components\Concerns\HasFileAttachments'
as follows:
--- /dev/null
+++ ../src/Components/Concerns/HasFileAttachments.php
@@ -117,17 +117,6 @@
return null;
}
if ($storage->getVisibility($file) === 'private') {
try {
return $storage->temporaryUrl(
$file,
now()->addMinutes(5),
);
} catch (Throwable $exception) {
// This driver does not support creating temporary URLs.
}
}
-
return $storage->url($file);
}
}
Database Engineering
SQLite Production Configuration I opted for SQLite to maintain simplicity, reliability and performance:
- Configured the database for optimal performance in a production environment
- Implemented proper indexing to speed up common queries
- Set up automated backup procedures twice a month to Cloudflare R2
For backups, I use a library called laravel-backup by Spatie. It will create a command
php artisan backup:run --only-db
that I can then call from my Coolify instance to back up the db to Cloudflare R2.
UI Development
Flux UI Customization I extended the Flux UI library to create a unique visual identity:
- Customized component styling while maintaining accessibility and keeping true to my Website design language.
Development Workflow
Automated Code Quality Tools I established a robust development workflow with:
- Pre-commit hooks for linting and formatting using Duster and Blade Formatter
- Static analysis with PHPStan to catch potential issues
- Type checking to prevent runtime errors
- Consistent coding standards enforced throughout the codebase
Self-Hosting & DevOps Implementation
I deployed my website on a self-managed VPS (from Hetzner) using Coolify, creating a professional-grade hosting environment.
Server Setup & Management
VPS Configuration I performed complete server setup, including:
- Secure SSH configuration with key-based authentication
- Firewall configuration to protect server resources
- Monitoring of VPS resources
Continuous Deployment
Coolify Implementation I established an automated deployment pipeline:
- Connected GitHub repository branches to Coolify for automatic builds
- Custom nixpacks.toml configuration for efficient builds on Coolify.
- Created deployment hooks for pre and post-deployment tasks
- Set up environment-specific configurations for staging and production
- Implemented health checks to verify successful deployments
Multi-Environment Configuration I created separate environments to ensure stability:
- Production environment tied to the main branch
- Staging environment for feature testing before release
- Development environment for local work
- Environment-specific environment variables and configurations
Analytics & Monitoring
Self-Hosted Analytics I deployed Umami for privacy-respecting analytics:
- Custom dashboard for monitoring site traffic and user behavior
- Event tracking for important user interactions
- Conversion tracking for engagement goals
- All analytics data stored on my own infrastructure for privacy
Server Monitoring I implemented comprehensive monitoring:
- Resource usage tracking (CPU, memory, disk)
- Performance metrics collection
- Log aggregation for troubleshooting
Outcomes and Learnings
This project significantly expanded my technical capabilities in modern web development. By building and deploying a production-quality personal website, I gained hands-on experience with the entire web application lifecycle from development to deployment and maintenance.
I believe that the skills developed through this project directly translate to professional environments where similar technologies and workflows are used to build scalable, maintainable web applications. The combination of Laravel backend solutions, modern frontend implementation, and DevOps knowledge demonstrates my ability to work across the full stack with professional-grade solutions.
Through challenges like optimizing for performance, implementing security best practices, and creating reliable deployment pipelines, I've developed problem-solving skills that will be very helpful throughout my whole career.